Originally posted on the Unreal Engine blog.
As you build larger projects with Blueprints, it’s easy to end up with an overwhelming sea of nodes. However, we’ve built in a number of different encapsulation and code reuse mechanisms to help you battle the chaos.
To encapsulate something is “to show or express the main idea or quality of (something) in a brief way”; in other words we can hide a complex sequence of nodes with a simple stand-in that conveys the same meaning or idea. You can still drill down and see how it works ‘under the hood’, but you don’t have to worry about the details when looking at the broader picture.
Code reuse is frequently paired along with encapsulation, but basically it’s the approach of applying the same idea (set of nodes) in different situations without having to duplicate your work each time. It’s obviously more effort to duplicate the work each time, and any bugs or feature changes would have to be done in each copy independently.
Here’s a quick comparison of the benefits and limitations of the different approaches:
Functions | Events | Macros | Collapsed Graphs | |
Execution Paths | One | One | Any | Any |
Non-exec Outputs | Any | None | Any | Any |
Latent actions | No | Yes | Yes | Yes* |
Add components/timelines | No | Yes | No | Yes* |
Other events | No | N/A | No | Yes* |
* A collapsed graph inherits the limits of the graph it is in, e.g., a collapsed graph inside of a function still cannot contain latent actions or timelines.
Collapsed Graphs
You can make a collapsed graph from a selection of nodes by right-clicking on one of the nodes and selecting ‘Collapse Nodes’. Any wires to nodes outside of the selection set will become inputs or outputs to the new collapsed node. Once collapsed, you can hover over the node to see a preview of the nodes in the contained graph.
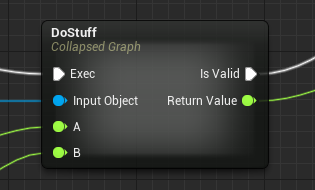
Collapsed graphs can contain any kind of node that is allowed in their current context and have no limits on their inputs/outputs. Collapsed graphs may seem like the best pick of the bunch with no limitations on the nodes they contain, but they are limited to only encapsulation, not code reuse. If you copy-paste a collapsed graph, all of the nodes inside get duplicated too, making a new collapsed graph rather than referencing the original.
Functions and Events
You can create your own empty functions using the Add Function button on the ‘My Blueprints’ toolbar or turn a selection of nodes (if it meets the limitations) into a function using the ‘Collapse to Function’ option when right-clicking on a selected node. You can make new events using the Add Custom Event… action in the graph context menu. A function shows up as a separate graph in the My Blueprints list, while events go into an event graph which can contain many different events.
Functions and events can both be called from other places in the blueprint just like calling a function defined in C++. Both of them define a single path of execution from an outside caller’s perspective, although they can have branching or loops internally. Events can also have their execution flow delayed by a latent action or even merge into the flow for another event.
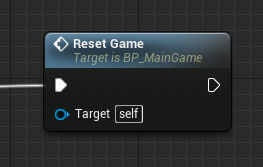
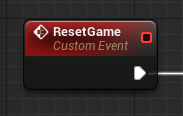
Functions are guaranteed to execute and return immediately by limiting what kinds of nodes can be placed in a function (latent actions, timelines, etc… are all prohibited). This allows them to return a value to a C++ caller, which isn’t possible when using events or macros.
Functions and events are also how C++ can call into Blueprints. C++ can define a function as a BlueprintImplementableEvent
which can be implemented in a Blueprint. These functions have no built-in behavior, and will do nothing if called without a blueprint implementation, but they can instead be declared as a BlueprintNativeEvent
, and provided with a C++ definition as well. Although these UFUNCTION()
keywords mention just events, they will turn into either events or functions in a blueprint when implemented in a Blueprint, depending on whether they have any return values or not.
Macros
You can create a new empty Macro using the Add Macro option on the ‘My Blueprints’ toolbar, or turn a selection of nodes into a macro using the ‘Collapse to Macro’ option when right-clicking on a selected node. You can also create Blueprint Macro Libraries, sharing macros across many blueprints. This makes them one of the most effective means of Blueprint code reuse. I’ll go into more depth on macros and macro libraries in a future post.
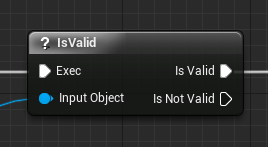
Macros can have arbitrary inputs and outputs, including execution wires. Macros can be called in the same way as functions or events from a blueprint, but they aren’t visible from C++ code. Like collapsed graphs, macros don’t really exist in a compiled blueprint; every instance gets expanded out into a unique set of nodes during compilation.
Since you can have more than one instance of a macro, some things like custom events are prohibited inside of the macro, since there’d be ambiguity in which one (or in which order) to execute them. Timelines and Add Component nodes are currently also prohibited due to implementation details, but we’d like to allow them in the future.
Comments
Comments are basically a present to future-you from current-you; explaining what your intentions were or making a note of potential issues and additional work to be done.
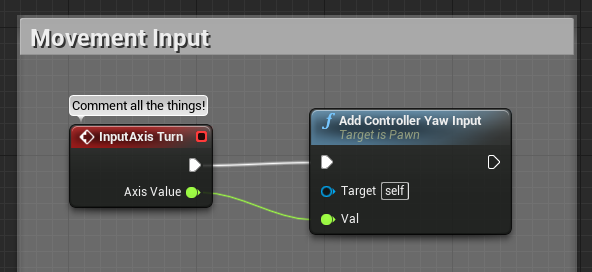
You can place a comment box around selected nodes by pressing C. By default these boxes move all contained nodes around with them, but you can change that behavior as well as the color in the Details panel. You can edit the comment just like any other editable title by double-clicking on it or pressing F2. You can also comment any other individual nodes using the field in the context menu for that node, which will show up as a bubble above the node.
Finally, you can write a comment that will show up in the Content Browser tooltip for your Blueprint by ‘Blueprint Properties’ and editing the ‘Blueprint Description’ in the Details panel. This is really useful for gameplay Blueprints that don’t have a thumbnail preview, but it’s still good to add usage notes even for things like level props that have a thumbnail.